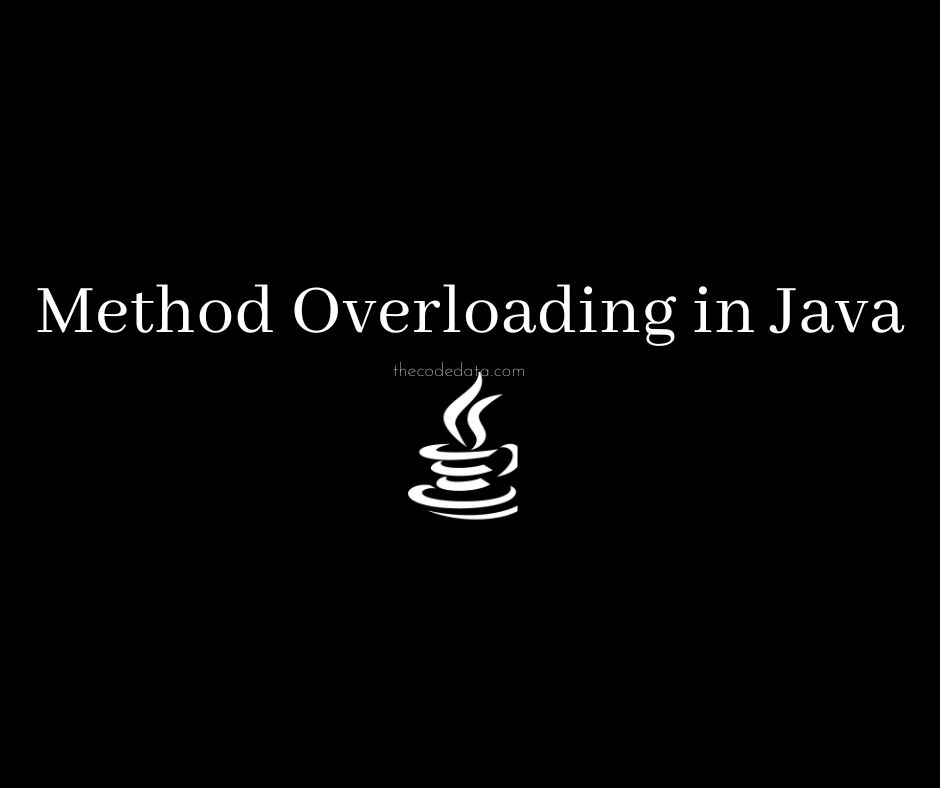
Two methods are said to be overloaded if and only if both methods have the same name but different argument types.
In the C language method, the overloading concept is not applicable, hence we cannot declare multiple methods with the same name and different argument types. If there is a change in argument type we must go for a new method name, it increases the complexity of programming.
But in Java, we can declare multiple methods with the same name but different argument types, such types of methods are called as overloaded methods.
Having an overloading concept in Java reduces the complexity of the programming.
public class MethodOverloading {
public void method(){
System.out.println("No- Argument");
}
public void method(int a){
System.out.println("Method with one argument");
}
public void method(int a, int b){
System.out.println("Method with two argument");
}
public static void main(String[] args) {
MethodOverloading object = new MethodOverloading();
object.method();
object.method(2);
object.method(1,2);
}
}

Note- In the overloading method resolution is always taken care of by the Java compiler based on reference type, hence overloading is also considered as compile4 time polymorphism or early binding.
Method Overloading Different Scenario1
Scenario1
Automatic promotion in overloading while resolving overloaded methods, If the exact match method is not available then we would not get any compile time error immediately first compiler will promote the argument to the next level and check whether the matched method is available or not, if the matched method will available then this method will be considered. If the matched method is not available then the compiler promotes the argument once again to the next level. This process will continue untill all possible promotions, still if the matched method is not available then we will get compile time error.
Following are the all possible promotion in overloading.
byte -> short -> int -> long -> float -> double
char -> int -> long -> float -> double
This process is called automatic promotion in overloading.
public class MethodOverloading {
public void method(int a) {
System.out.println("int-arg");
}
public void method(float f) {
System.out.println("float-arg");
}
public static void main(String[] args) {
MethodOverloading object = new MethodOverloading();
object.method('A'); // char will be promoted to int data type
object.method(5l); // long will be promoted to float argument
object.method(1); // there is no promotion require
}
}

Note- While resolving an overloaded method compiler will always give precedence to the child type argument in comparison with parent type argument.
Scenario2
The var-args method will get the least priority that is if there is no other method matched then only the var-args method will get a chance. It is the same as the default case in the switch statement in Java.
public class MethodOverloading {
public void method(int a) {
System.out.println("int-arg");
}
public void method(int... x) {
System.out.println("var-arg");
}
public static void main(String[] args) {
MethodOverloading object = new MethodOverloading();
object.method();//Var-arg method will be called
object.method(1); //int argument method will be called
object.method(1,2); // Var-arg method will be called
}
}

Similar Java Tutorials
- Bitwise operator in Java
- Operators in Java
- Public static void main
- Command line argument in Java
- Java coding standards
- Control Flow Statements in Java
- If – else in Java
- Switch statements in Java
- For loop in Java
- While loop in Java
- Do while loop in Java
- Import statement in Java
- Abstract modifier in Java
- Strictfp modifier in Java
- Final variable in Java
- Static modifier in Java
- Native modifier in Java
- Transient keyword in Java
- Volatile keyword in Java
- Adapter Class in Java
- Method signature in Java
- Method overloading in Java
- Has A Relationship
- Inheritance in Java
- Encapsulation in Java
- Abstraction in Java
- Data Hiding in Java
- Interface vs abstract class
9 thoughts on “Method Overloading in Java”