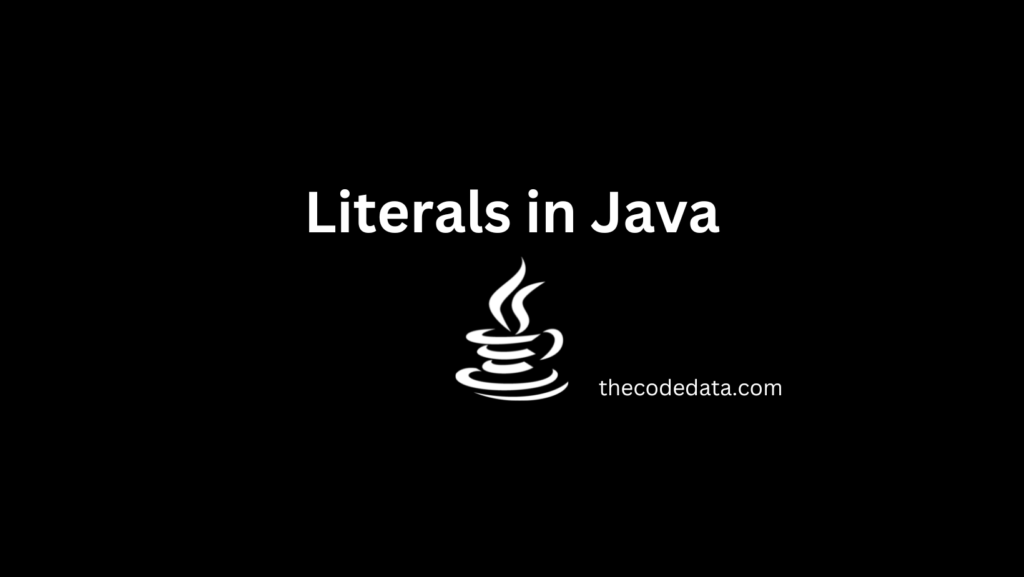
Literals:-
A constant value which can be assigned to the variable is called literals. Java has various type of literals.
Example:-

Integral Literals:-
For integral data type( byte, short, int, long). We can specify literal values in the following ways.
1. Decimal Literals:-
In Decimal literals allowed digits are 0 to 9. It can be positive or negative.
int x = 12; (Decimal Form).
2. Octal Literals:-
In Octal literals values should be prefix with 0. In octal literal allowed digits are 0 to 7.
Example:–
int x = 012;
3. Hexa Decimal Literals:-
In Hexa Decimal literals allowed digits are 0 to 9 and characters are a to f( 10 to 15). we can use both lower case and upper case.
Example:–
int x = 0X10;
There are only possible ways to specify literal values for integral data type.
int a = 20;
int a = 0724; ( Integer value to large)
int a = 0777;
int a = 0Xface;
int a = 0XDef;
Int x = OXFare ( r not allowed)
By default every integral literal is of int type. But we can specify explicitly as long type by suffixed with small or capital L .
Example:-
int x = 100; (Valid)
long l = 10L; (valid)
int x = 10L; (invalid)
Floating Point Literals:-
By default every floating point literals is of double type and hence we can’t assign directly to the float variable. But we can specify floating point literals float type by suffixed with small or capital F.
Example:-
float f = 123.456F;
We can specify floating point literals only in decimal form and we can’t specify in octal and hexadecimal form.
Example:-
double d = 123.456; (valid)
double d = 098.321; (valid)
double d = 0x 123.543 (Invalid)
We can specify floating point literals even in exponential form (scientific form).
Example:-
float f = 1.2e3F;
Boolean Literals:-
Only true or false values are allowed for Boolean literals.
Example:-
boolean b = true; (valid)
boolean b =0; (invalid)
Char Literals:-
We can specify char literals as a single character within single coots. Without single coots, it shows an error cannot find symbol.
Example:-
char ch = 'a'; (valid)
char ch = 'aa'; (invalid)
We can specify char literals as UNICODE Literals. which represents the UNICODE value of that character and integral literals can be specified either in decimal, octal, or hexadecimal forms but the allowed range is 0 to 65535.
Example:-
char ch = 99;
Output will be ‘c’ because the UNICODE value of 99 is ‘c’.
- Every Escape character is a valid char literal.
Example:-
char ch = '\n';
char ch = '\t';
char ch = \b';
String Literals:-
- Any sequence of character with in double coots is treated as string literal.
Example:-
String s = "java";
- we can specify literal values even in binary form allowed digits are 0 and 1. The literal value should be prefixed with 0b or 0B.
Example:-
int x = 0B1111;
- We can use the underscore ‘_’ between digits of numeric literals.
Example:-
double d = 232112.962;
double d = 2_32_112.9_6_2 (Here we use underscore)
The main advantage of this approach is the readability of code will be improved. At the time of compilation, these underscore symbols will be removed automatically. We can use more than one underscore symbol also between the digits. We can use underscore only between the digits. if we use it anywhere else we will get compile time error.
11 thoughts on “Literals in Java”