In this article, we are going to create a simple project using gRPC & java. It’s a complete beginner-level project using gRPC and Java
What is gRPC ??
Before going towards code let’s know about gRPC. So gRPC is an open-source RPC framework created by Google. You can make projects with gRPC by using any programming language like C++, Python, Java, Go, and many more. gRPC is not dependent on any language. You can explore more about gRPC by using their official site https://grpc.io/
Steps to develop any project or service using gRPC
Create proto file and generate classes in your language
A proto file is written by using proto buffer. A proto file consists of all endpoints of services along with request and response messages. After creating the proto file, now need to generate proto Stubbs using the ProtoC compiler. for exploring more about proto buffer and proto file you can visit the official site of proto buffer https://developers.google.com/protocol-buffers
Implement your logic in your language
After generating stubs or classes in your language now you have to write all your logic and operation by extending your service classes
Create Server and host your Service
At the end you have to create a server and add your all service classes and server
Let’s start project development using gRPC and Java
Here we are going to use Maven for developing our product, in maven we can add dependencies and plugin.
Maven dependencies for gRPC
We have to add following dependencies in pom.xml file
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>1.49.0</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.49.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.49.0</version>
</dependency>
<dependency>
<groupId>org.apache.tomcat</groupId>
<artifactId>annotations-api</artifactId>
<version>6.0.53</version>
<scope>provided</scope>
</dependency>
After these dependencies we also need to add following plugin for compiling our proto file
<plugin>
<groupId>com.github.os72</groupId>
<artifactId>protoc-jar-maven-plugin</artifactId>
<version>3.11.1</version>
<executions>
<execution>
<phase>generate-sources</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<includeMavenTypes>direct</includeMavenTypes>
<inputDirectories> <include>src/main/</include></inputDirectories>
<outputTargets>
<outputTarget>
<type>java</type>
<outputDirectory>src/main/java</outputDirectory>
</outputTarget>
<outputTarget>
<type>grpc-java</type>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.15.0</pluginArtifact>
<outputDirectory>src/main/java</outputDirectory>
</outputTarget>
</outputTargets>
</configuration>
</execution>
</executions>
</plugin>
Let’s start coding
This is our final project structure
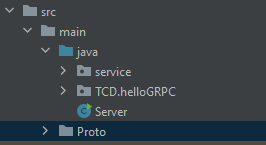
Proto file
Inside main folder create a folder named Proto and inside this folder create a file and named it Hello.Proto
Here we are going to create two endpoints, one is hello and another is bye. Also we need to write request and response messages
Hello.Proto
syntax="proto3";
package TCD.helloGRPC;
option java_multiple_files = true;
service helloGRPC{
rpc Hello( request) returns (response);
rpc Bye( request) returns (response);
}
message request{
string name=1;
}
message response{
string reply=1;
}
after writing proto file we can generated classes or stubbs by using mvn command because we already added dependencies and plugin for gRPC and proto file.
mvn clean install
After executing the mvn clean install you can see that some code is generated in the java folder,a package named ‘gfc.helloGRPC’ is created, inside that, you will find many classes which are generated from the proto file. These generated codes are in Java language but we can also generate these codes in any other programming language like python, C++, Go, etc.
This is the list of all classes generated from ‘Hello.proto’ file
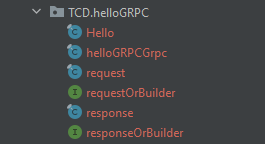
after this, we need to create a new java class ‘ServiceImpl’ by extending the ‘helloGRPCImplBase’ class generated by the protoc compiler. Inside this ‘ServiceImpl’ class, we can write our logic for all the endpoint inside our service in the proto file
ServiceImpl.java
package service;
import TCD.helloGRPC.helloGRPCGrpc;
import TCD.helloGRPC.request;
import TCD.helloGRPC.response;
import io.grpc.stub.StreamObserver;
public class ServiceImpl extends helloGRPCGrpc.helloGRPCImplBase {
@Override
public void hello(request request, StreamObserver<response> responseObserver) {
String name = request.getName();
response response = TCD.helloGRPC.response.newBuilder().setReply("Hii "+name).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
@Override
public void bye(request request, StreamObserver<response> responseObserver) {
response response = TCD.helloGRPC.response.newBuilder().setReply("Bye "+request.getName()+"!!").build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
now we have to create server to start our service , for that we need to create a server. for this create a class named Server
Server.java
import io.grpc.ServerBuilder;
import service.ServiceImpl;
import java.io.IOException;
public class Server {
public static void main(String[] args) throws IOException, InterruptedException {
int port = 8000;
io.grpc.Server server = ServerBuilder.forPort(port).addService(new ServiceImpl()).build().start();
System.out.println("server started at port: "+port );
server.awaitTermination();
}
}
Test the gRPC end points
now just run this Server class and test our endpoints. we can test with bloomRPC, postman, or any other client. for checking the working of our project in bloomRPC you can watch this video
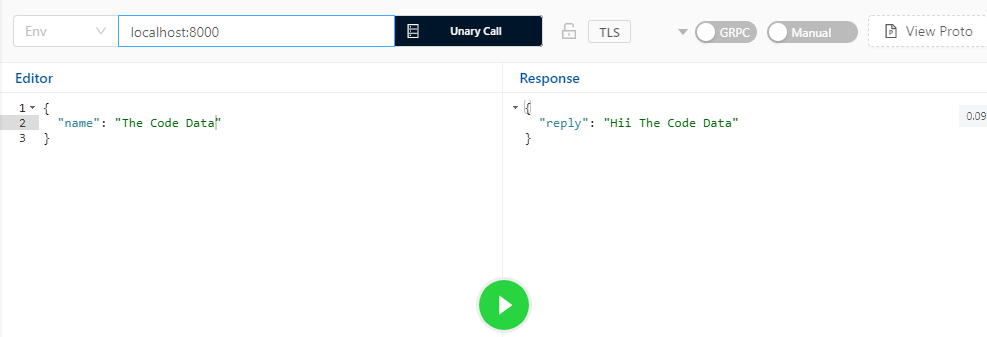
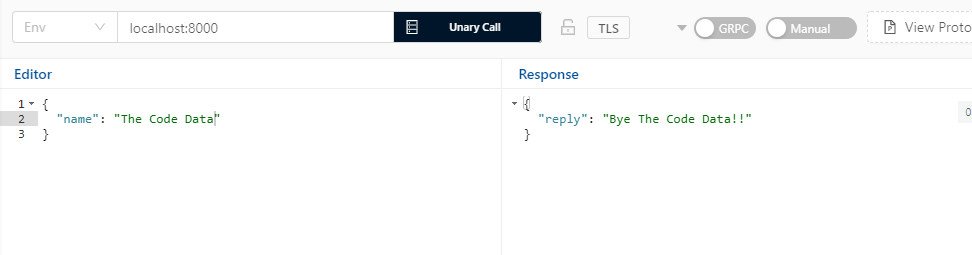
this is a small project by using gRPC and Java, for more projects and articles related to coding and programming you can visit our site and you tube channel
thanks
Download this grpc java project
This project is available on the git hub you can download it from there through this link https://github.com/gangforcode/grpc-java-Hello
3 thoughts on “gRPC with Java”