In this article, we are going to write a simple java program to sorts numbers in ascending order. Don’t worry if you new to programming in this article every step is break down to make it easy to understand.
Sorting in Ascending Order
Imagine you have a bunch of numbers in a list, and you want to arrange them in increasing order. For example, if you have the numbers 5, 2, 9, 1, and 3, sorting them in ascending order would result in 1, 2, 3, 5, and 9.
Java Code for Sorting Numbers in Ascending Order : Bubble Sort
Let’s write a Java Program to Sort Number in Ascending Order. Here we will use Bubble Sort. The Algorithm repeatedly steps through the list, compare adjacent elements, and swaps them if they are in the wrong order. The process is repeated for each element until entier list is sorted.
public class BubbleSort {
public static void main(String[] args) {
int[] numbers = {11,23,1,54,7,87,23,98,23};
for(int i=0;i<numbers.length-1;i++){
for(int j=0;j<numbers.length-1-i;j++){
if(numbers[j]>numbers[j+1]){
int temp = numbers[j];
numbers[j]= numbers[j+1];
numbers[j+1]= temp;
}
}
}
System.out.println("Numbers in Ascending Order");
for(int number:numbers){
System.out.print(number+" ");
}
}
}
Code Explenation
- We started with an Array of unsorted number named
numbers
- The outer for loop is used for interating the array, each time moving the largest number to its correct position.
- The inner for loop compare the adjecent elements and swaps if they are in wrong order.
- Swaping is performed using temparory variable
temp
. - Finally, we printed the sorted array using for each loop
Output
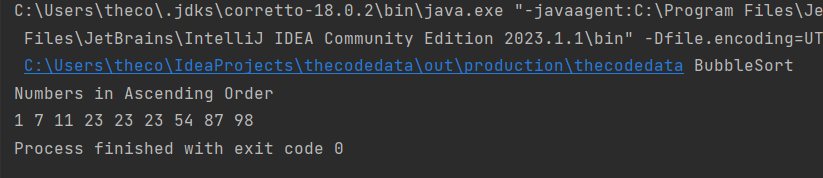