synchronized is a modifier in Java, Only applicable for methods and blocks but not for classes and variables.
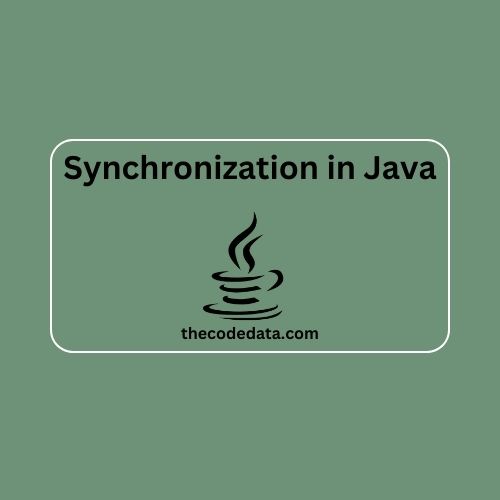
If multiple Threads are trying to operate on the same Java object simultaneously then there is a chance of data inconsistency problem. To overcome this problem we should go for a synchronized keyword.
If a method or block is declared as synchronized then only one Thread is allowed to execute that block or method and given object at a time, so that the data inconsistency problem will be resolved.
The main advantage of synchronized keyword is that we can resolve the time consistency problem but there is a main disadvantage of synchronized keyword is that it increases waiting time and create a performance problem. hence there is no specific requirement then it is not recommended to use synchronized keywords.
The internally synchronized concept is implemented by using the lock
. Every object in Java has a unique lock so whenever we use a synchronized keyword then only the lock concept is used,
If a thread wants to execute a synchronized method on the given object first it has to get a lock
of that object. Once the e thread got the lock then the thread is allowed to execute only the synchronized method on that object once method execution is completed the Thread releases the lock automatically.
Acquiring and releasing lock is internally performed by JVM, we as a programmer are not responsible for this activity.
While a Thread executes a synchronized method on the given object then remaining Threads are not allowed to execute any synchronized method simultaneously on the same object but remaining Threads are allowed to execute non-synchronized methods simultaneously.

The lock concept is implemented based on the object but not based on the method.
Synchronized Block Declration
We can declare synchronized block as follows
- To get lock of current object
synchronized(this){
//
//
//
}
If a Thread gets a lock of a current object then only it is allowed to perform execute this area.
- To get lock of particular object ‘b’
synchronized(b){
//
//
//
}
If a Thread gets a lock of a particular object then only it is allowed to perform execute this area.
- To get class level lock
synchronized(class){
//
//
//
}
If a Thread gets class level lock then only it is allowed to execute this area.
public class Display {
public void wish (String name){
synchronized (this){
for(int i=0;i<10;i++){
System.out.println("Good Morning");
try{
Thread.sleep(2000);
}
catch (InterruptedException e){
System.out.println("Name");
}
}
}
}}
class MyThread extends Thread{
Display d;
String name;
MyThread(Display d, String name){
this.d = d;
this.name = name;
}
@Override
public void run() {
d.wish(name);
}
}
class SynchronizedDemo{
public static void main(String[] args) {
Display d = new Display();
MyThread t1 = new MyThread(d , "yuvraj");
MyThread t2 = new MyThread(d , "dhoni");
t1.start();
t2.start();
}
}
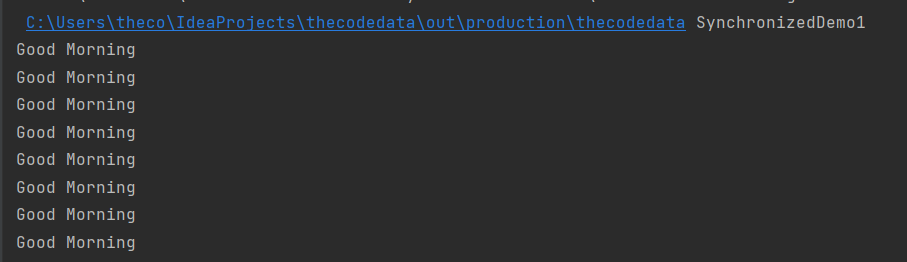
Similar Java Tutorials
- Odd Even in Java
- Method to print exception information in Java
- Exception Handling by Using try-catch in Java
- Checked Exception vs Unchecked Exception
- Exception Hierarchy in Java
- Java Exception Handling Interview Questions
- final finally finalize in java
- User Defined Exception in Java
- Exception Handling Keyword in Java
- throw throws in Java
- try with multiple catch block in Java
- Threads in Java
- Thread priority in Java