Data Structures are very important in the world of programming to manage and organize data efficiently one such Data structure is HashSet, HashSet is a versatile and powerful collection that offers unique features to manage and organize data.
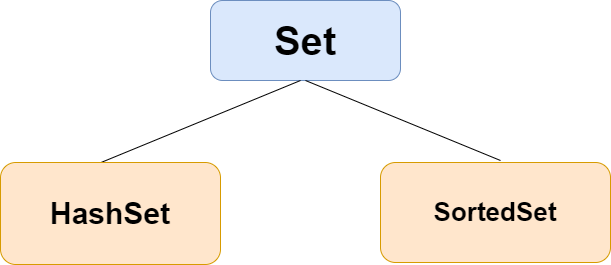
What is HashSet?
HashSet is a part of the Java Collections Framework and is available in Java.util
package and implement the Set interface. It is known for it’s exceptional performance when it comes to adding, removing, and checking the elements. It is an unordered collection and duplicate values are not allowed.
Properties of HashSet
- Hash Table is the underline data structure.
- Insertion order is not preserved. Insertion order is based on the HashCode of the object.
- Duplicate objects are not allowed.
- Heterogeneous objects are allowed.
- Null insertion is allowed but at most one time.
- HashSet implements java.util.Set, Cloneable, java.io.Serializable.
Note- In HashSet duplicates are not allowed but if we try to insert any duplicate then we will not get any compile time and run time error instead of error add() method returns false.
import java.util.HashSet;
public class HashSetDemo {
public static void main(String[] args) {
HashSet hSet = new HashSet();
System.out.println(hSet.add("Java"));
System.out.println(hSet.add("Java"));
}
}

HashSet Constructor
HashSet()
HashSet hSet = new HashSet();
This constructor creates an empty HashSet object with a default initial capacity of 16 and a default fill ratio/ load Factor of 0.75.
HashSet(int initialCapacity )
public HashSet( @Range(from = 0, to = Integer.MAX_VALUE) int initialCapacity )
This constructor creates an empty HashSet object with a specified initial capacity and default fill ratio/load Factor of 0.75.
HashSet( initialCapacity, float loadFactor )
public HashSet( @Range(from = 0, to = Integer.MAX_VALUE) int initialCapacity,
float loadFactor )
This constructor creates an empty HashSet object with a specified initial capacity and specified fill ratio/load Factor.
HashSet( Collection c )
public HashSet( @NotNull Collection<? extends E> c )
This constructor creates an equivalent HashSet for the given collection. This constructor is used for interconversion between collection objects.
HashSet Methods
add()
public boolean add( E e )
We can use the add()
method to add elements in the HashSet
remove()
public boolean remove( Object o )
this method removes specified Object from HashSet
contains()
public boolean contains( Object o )
This method returns true if a specified object is present in the HashSet.
Itterating HashSet elements
We can easily iterate HashSet elements by using for – each loop.
for (String element:hSet){
System.out.println(element);
}
HashSet Java Example
import java.util.HashSet;
public class HashSetDemo {
public static void main(String[] args) {
HashSet<String> hSet = new HashSet(2 );
hSet.add("The"); // adding element
hSet.add("Code"); // adding element
hSet.add("Data"); // adding element
hSet.add("java"); // adding element
hSet.remove("java"); // removing element
System.out.println(hSet.contains("Data")); // checking if element exist or not
// iterating HashSet elements
for (String element:hSet){
System.out.println(element);
}
}
}

6 thoughts on “HashSet in Java: Complete Tutorial”